Примеры моделирования дискретных схем на VHDL
В качестве примера приведем проекты на VHDL, реализующие при помощи различных форм VHDL-описаний простейший одноразрядный сумматор с учетом переноса (см. рисунок), а также сумматор с произвольной разрядностью.
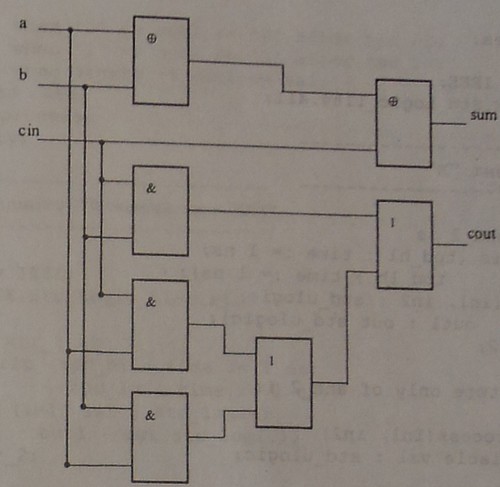
Двоичный одноразрядный сумматор с переносом
Определим необходимые элементы для реализации одноразрядного сумматора с переносом — это элементы and, or и xor. Реализуем данные элементы в виде библиотеки gates:
library IEEE;
use IEEE.std_logic_1164.all;package gates is
component and_2
generic (tpd_h1 : time := 1 ns;
tpd_lh : time :=1 ns);
port (in1, in2 : std_ulogic;
out1 : out std_ulogic);
end component;component or_2
generic (tpd_h1 : time := 1 ns;
tpd_lh : time := 1 ns);
port (in1, in2 : std_logic;
out1 : out std_logic);
end component;component xor_2
generic (tpd_hl : time := 1 ns;
tpd_lh : time := 1 ns);
port (in1, in2 : std_logic;
out1 : out std_logic);
end component;
end gates;library IEEE;
use IEEE.Std_Logic_1164.all;
—————————
— Элемент "И"
—————————
entity and_2 is
generic (tpd_hl : time := 1 ns;
tpd_lh : time := 1 ns);
port (in1, in2 : std_ulogic;
out1 : out std_ulogic);
end and_2;architecture only of and_2 is
begin
p1: process (in1, in2)
variable val : std_ulogic;
begin
val := in1 and in2;
case val is
when ‘0’ => out1 <= '0' after tpd_hl; when '1' => out1 <= '1' after tpd_lh; end case; end process; end only; ------------------------- -- Элемент "ИЛИ" -------------------------- library IEEE; use IEEE.Std_Logic_1163.all; entity or_2 is generic (tpd_hl : time := 1ns; tpd_lh : time := 1ns); port (in1, in2 : std_logic); out1 : out std_logic); end or_2; architecture only of or_2 is begin p1: process (in1, in2) variable val : std_logic; begin val := in1 or in2; case val is when '0' => out1 <= '0' after tpd_hl; when '1' => out1 <= '1' after tpd_lh; when others => out1 <= val; end case; end process; end only; -------------------------- -- Элемент "Исключающее ИЛИ" -------------------------- library IEEE; use IEEE.Std_Logic_1164.all; entity xor_2 is generic (tpd_hl : time := 1ns; tpd_lh : time := 1ns); port (in1, in2 : std_logic); out1 : out std_logic); end xor_2; architecture only of xor_2 is begin p1: process (in1, in2) variable val : std_logic; begin val := in1 xor in2; case val is when '0' => out1 <= '0' after tpd_hl; when '1' => out1 <= '1' after tpd_lh; when others => out1 <= val; end case; end process; end only;
Реализуем простейший сумматор с учетом переноса на поведенческом и структурном уровнях, используя логические элементы из библиотеки
gates:
library IEEE;
use IEEE.Std_Logic_1164.all;entity simple_adder is
port (a : in std_logic;
b : in std_logic;
cin : in std_logic;
sum : out std_logic;
cout : out std_logic);
end simple_adder;— Описание простейшего сумматора при помощи
— параллельного присваивания (потоковая форма)architecture rtl of simple_adder is
begin
sum <= (a xor b) xor cin; cout <= (a and b) or (cin and a) or (cin and b); end rtl; -- Описание простейшего сумматора на -- структурном уровне -- Используем описания из библиоткеи gates use work.gates.all; architectrue structural of simple_adder is signal xor1_out, and1_out, and2_out, or1_out : std_logic; begin xor1: xor_2 port map (in1 => a, in2 => b, out1 => xor1_out);
xor2: xor_2 port map (in1 => xor1_out, in2 => cin, out1 => sum);
and1: and_2 port map (in1 => a, in2 => b, out1 => and1_out);
or1: or_2 port map (in1 => a, in2 => b, out1 => or1_out);
and2: and_2 port map (in1 => cin, in2 => or1_out, out1 => and2_out);
or2: or_2 port map (in1 => and1_out, in2 => and2_out, out1 => cout);
end structural;
В качестве примера использования настройки приведем реализацию сумматора с последовательным переносом с произвольной (определяемой пользователем) разрядностью, в котором в структурном описании используется разработанный ранее простейший сумматор:
—————————
— N-битный сумматор
— Размерность сумматора определяется
— параметром настройки N
—————————library IEEE;
use IEEE.Std_Logic_1164.all;entity adderN is
generic(N: integer := 16);
port (a : in std_logic_vector (N downto 1);
b : in std_logic_vector (N downto 1);
cin : in std_logic;
sum: out std_logic_vector (N downto 1);
cout : out std_logic);
end adderN;— Реализация сумматора на структурном уровне
architecture structural of adderN is
component simple_adder
port (a : in std_logic;
b : in std_logic;
cin : in std_logic;
sum : out std_logic;
cout : out std_logic);
end component;
signal carry : std_logic_vector (0 to N);
begin
carry (0) <= cin; cout <= carry (N); -- Конкретизируем простейший сумматор N раз gen: for I in 1 to N generate add: simple_adder port map ( a => a (I),
b => b (I),
cin => carry (I-1),
sum => sum (I),
cout => carry (I));
end generate;
end structural;— Реализация сумматора на поведенческом уровне
architecture begavioral of adderN is
begin
p1: process (a, b, cin)
variable vsum: std_logic_vector (N downto 1);
variable carry: std_logic;
begin
carry := cin;
for i in 1 to N loop
vsum (i) := (a(i) xor b(i)) xor carry;
carry := (a(i) and b(i)) or (carry and (a(i) or b(i)));
end loop;
sum <= vsum; cout <= carry; end process p1; end behavioral;